JavaScript Functions Explained: A Simple Tutorial for Beginners
Learn the basics of JavaScript functions in this beginner-friendly tutorial. Understand how to define, use parameters, return values, and explore key concepts like function expressions and arrow functions to improve your coding skills.

JavaScript is a versatile and powerful programming language that powers much of the interactive web. Whether you're building websites, web apps, or adding dynamic behavior to pages, mastering JavaScript is crucial. One of the key concepts you'll encounter early on in your JavaScript learning journey is functions. Functions are fundamental building blocks of JavaScript, and understanding them is essential for writing clean, maintainable code.
In this beginner-friendly tutorial, we will explore JavaScript functions, breaking down what they are, how they work, and why they are so important. This guide is designed for those who are new to programming or are just starting with JavaScript. By the end, you'll have a solid understanding of how to define and use functions in your code.
What Is a Function in JavaScript?
At its core, a function in JavaScript is a reusable block of code designed to perform a specific task. Instead of writing the same code over and over again, you can place it inside a function and call that function whenever you need it. Functions help organize and structure your code, making it more efficient and easier to maintain.
Functions allow you to break complex tasks into smaller, manageable pieces. This not only makes your code more readable but also helps reduce redundancy. For example, instead of repeating the same code to calculate a result multiple times, you can define a function to handle the calculation and simply call it whenever needed.
Why Are Functions Important?
Functions are important because they bring several advantages to your code:
1. Reusability: You can define a function once and call it multiple times. This eliminates the need to repeat code, which can save time and reduce the likelihood of errors.
2. Modularity: Functions allow you to divide your code into smaller, self-contained units that each perform a specific task. This makes your code easier to debug, maintain, and scale as your project grows.
3. Clarity and Readability: By grouping related tasks inside a function, your code becomes more organized. Other developers (or even you, in the future) can quickly understand what each function does without having to read through lines of code.
Defining a Function
In JavaScript, defining a function involves creating a function declaration. A function declaration typically includes a name, followed by parentheses and a block of code. The name is used to refer to the function when calling it.
Functions can also take inputs, known as parameters, which allow you to pass values into the function for processing. The function then performs a specific task using those inputs. For example, you could pass numbers into a function that adds them together and returns the result.
Parameters and Arguments
When you define a function, you often include parameters in the parentheses. These parameters are placeholders for the values that will be passed to the function when it is called. Parameters are like variables that exist within the function.
When calling a function, you provide arguments, which are the actual values that will replace the parameters inside the function. This allows functions to be dynamic and work with different inputs each time they're called.
For example, a function might calculate the total price of items in a shopping cart. By passing the price of each item as an argument, the function can calculate the total for different shopping carts with varying items.
Returning Values from Functions
One of the most powerful features of functions is the ability to return a value. This means that after the function has completed its task, it can send a result back to the part of the program that called it. This return value can then be used in other parts of the program, making functions highly useful for complex calculations or decision-making.
For instance, if you create a function to check if a user is eligible for a discount, it could return true
or false
based on certain conditions. This value could then be used elsewhere in your application to trigger actions like applying the discount or notifying the user.
Function Expressions and Arrow Functions
While traditional functions in JavaScript are defined using a function
keyword, there are also function expressions. A function expression allows you to define a function as part of a larger expression, often assigned to a variable. This can be particularly useful when working with callbacks, or when you want to pass functions around as arguments.
In addition, arrow functions, introduced with ES6 (ECMAScript 2015), provide a more concise way to define functions. Arrow functions are shorter and eliminate the need for the function
keyword. They also have unique behaviors related to how they handle this
keyword, which is important when working with objects or event handlers.
The Scope of Functions
Another concept to understand when working with JavaScript functions is scope. Scope refers to where a variable or function is accessible within the code. Functions can create their own scope, meaning that variables declared inside a function are only accessible within that function.
This concept is critical for avoiding conflicts between different parts of your program and ensuring that your functions don't inadvertently affect one another.
Conclusion
JavaScript functions are essential for writing clean, reusable, and maintainable code. They allow developers to break complex problems into smaller, manageable tasks, making code easier to read and debug. Functions also promote reusability and modularity, two key aspects of writing efficient code.
In this tutorial, we’ve covered the basics of functions in JavaScript, including how to define them, use parameters and arguments, return values, and the differences between function expressions and arrow functions. Understanding functions is a crucial step in your JavaScript learning journey, and as you continue to build more complex applications, you’ll find that functions are indispensable tools for solving problems and structuring your code effectively.
What's Your Reaction?

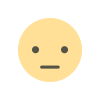

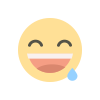


