How to Use TypeScript with Node.js Comprehensive Technical Guide
Learn how to use TypeScript with Node.js in this comprehensive technical guide. Discover step-by-step setup, best practices, and advanced tips to enhance your Node.js development with TypeScript I How to Use TypeScript with Node.js (Comprehensive Technical Guide)
Introduction
What is TypeScript?
JavaScript is expanded upon by TypeScript, a strongly typed programming language, which adds static types. It is compatible with any JavaScript runtime and is created and maintained by Microsoft. It compiles to ordinary JavaScript.
Why Use TypeScript with Node.js?
Node.js runs on the server side and provide a runtime environment for running JavaScript code. Dynamic typing is JavaScript; TypeScript adds static typing that increases maintainability, error protection, and code quality.
TypeScript's Principal Advantages in Node.js
- Type Safety: By identifying problems early in development, it lowers runtime errors.
- Improved Code Maintainability: Managing large codebases is made simpler.
- Enhanced IDE Support: VS Code and other editors support auto-completion, refactoring, and documentation.
- Scalability: Perfect for large-scale applications that need intricate structures.
Setting Up the Development Environment
Installing Node.js and TypeScript
First, ensure you have Node.js installed:
node -v npm -v
If not installed, download from Node.js official site.
Then, install TypeScript globally:
npm install -g typescript tsc -v
Initializing a Node.js Project
Create a new project folder and initialize package.json:
mkdir typescript-node-app && cd typescript-node-app npm init -y
Installing TypeScript and Dependencies
npm install --save-dev typescript
Configuring tsconfig.json
Generate a TypeScript config file:
tsc --init
Update tsconfig.json with
{ "compilerOptions": { "target": "ES6", "module": "CommonJS", "outDir": "./dist", "rootDir": "./src", "strict": true } }
Writing and Compiling TypeScript in Node.js
Writing and Compiling TypeScript in Node.js
Create a src folder and a index.ts file inside:
const greet = (name: string): string => { return `Hello, ${name}!`; }; console.log(greet("MoheedDev"));
Compiling TypeScript to JavaScript
tsc
It generates a dist/index.js
file.
Running TypeScript Files Directly
Instead of compiling manually, use ts-node
:
npm install -g ts-node ts-node src/index.ts
Using TypeScript with Express.js
Installing Express and Type Definitions:
npm install express npm install --save-dev @types/express
Creating an Express Server in TypeScript:
import express, { Request, Response } from "express"; const app = express(); const port = 3000; app.get("/", (req: Request, res: Response) => { res.send("Hello from TypeScript + Express!"); }); app.listen(port, () => { console.log(`Server running on http://localhost:${port}`); });
Middleware and Request Validation with TypeScript
Using body-parser
and custom middleware:
import express from "express"; import bodyParser from "body-parser"; const app = express(); app.use(bodyParser.json()); app.post("/data", (req, res) => { const { name }: { name: string } = req.body; res.send(`Received: ${name}`); }); app.listen(3000, () => console.log("Server running on port 3000"));
TypeScript Features for Node.js Development
Type Annotations and Interfaces:
interface User { id: number; name: string; } const user: User = { id: 1, name: "MoheedDev" }; console.log(user);
Working with Modules in TypeScript
Create utils.ts
:
export const add = (a: number, b: number): number => a + b;
Use it in index.ts
:
import { add } from "./utils"; console.log(add(5, 3));
Using Decorators in TypeScript:
function Log(target: any, propertyKey: string, descriptor: PropertyDescriptor) { console.log(`Method called: ${propertyKey}`); } class Example { @Log show() { console.log("Hello from decorator MoheedDev "); } }
Debugging & Error Handling in TypeScript
Debugging TypeScript in Node.js
Use --inspect
with ts-node
:
node --inspect dist/index.js
Common TypeScript Errors in Node.js
- Cannot find module: Fix by ensuring
tsconfig.json
includes"moduleResolution": "Node"
. - Type mismatch: Ensure function parameters and return values match expected types.
Error Handling Best Practices
Using try...catch
:
try { throw new Error("Something went wrong!"); } catch (error) { console.error("Error:", error.message); }
Testing a TypeScript Node.js Application
Setting Up Jest for TypeScript
npm install --save-dev jest ts-jest @types/jest
Configure jest.config.js
:
module.exports = { preset: "ts-jest", testEnvironment: "node", };
Writing Unit Tests for TypeScript Functions
const sum = (a: number, b: number): number => a + b; export { sum };
Test case:
import { sum } from "./sum"; test("adds 1 + 2 to equal 3", () => { expect(sum(1, 2)).toBe(3); });
Running Tests
npm test
Deployment & Best Practices
Using Webpack & Babel for Optimization
Install Webpack and Babel:
npm install --save-dev webpack webpack-cli babel-loader @babel/core @babel/preset-env
Update webpack.config.js
:
module.exports = { entry: "./src/index.ts", output: { filename: "bundle.js", path: __dirname + "/dist", }, module: { rules: [{ test: /\.ts$/, use: "babel-loader" }], }, resolve: { extensions: [".ts", ".js"], }, }; /*Console.log"moheeddev.com"*/
How to Deploy a TypeScript-Based Node.js App
( How to Use TypeScript with Node.js (Comprehensive Technical Guide) )
- Compile TypeScript to JavaScript
- Use
pm2
for process management
pm2 start dist/index.jspm2 start dist/index.js
Performance Optimization Tips
- Use
async/await
for efficient execution - Enable caching for repeated operations
- Minimize dependencies
Conclusion
This guide covers everything you need to integrate TypeScript with Node.js efficiently. Implement these best practices and debugging techniques to write scalable and maintainable backend applications.
For Watching a Full Article Click Here
If You are Interested this topics to Click Here this link:
Most Important JavaScript Frameworks to Study in 2025
What's Your Reaction?

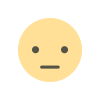

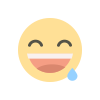


