Exploring Object-Oriented Programming with C++: A Step-by-Step Tutorial
C++ is a powerful, versatile programming language widely used in software development, game design, and systems programming. One of the core paradigms that C++ offers is Object-Oriented Programming (OOP). Understanding OOP in C++ is essential for creating efficient, reusable, and maintainable software. In this tutorial, we will explore the fundamentals of Object-Oriented Programming in C++, focusing on key concepts such as classes, objects, inheritance, polymorphism, and encapsulation.

This guide is intended for those who are familiar with programming concepts and are looking for the best C++ tutorial to deepen their understanding of OOP principles in C++. By the end of this article, you will have a comprehensive understanding of how to apply OOP techniques using C++.
What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm that uses objects and classes to organize code. It allows you to model real-world entities and their behaviors. At the heart of OOP are four main principles:
- Encapsulation – Bundling data and methods that operate on that data within a single unit or class.
- Abstraction – Hiding the complex implementation details and showing only the essential features of an object.
- Inheritance – Creating new classes from existing ones, inheriting properties and behaviors.
- Polymorphism – The ability to take multiple forms, allowing methods or objects to act in different ways based on context.
Now, let’s explore these concepts in C++.
Step 1: Understanding Classes and Objects
In C++, a class is a blueprint for creating objects. An object is an instance of a class. Think of a class as a template for creating objects, where the objects represent specific instances that have attributes and behaviors defined by the class.
Class – A user-defined type that represents a set of properties and behaviors. It serves as the foundation of an object.
Object – An instance of a class containing specific data and performing actions defined in the class.
For example, imagine a class called Car. The Car class might have properties like color, make, and model, and behaviors like accelerate() and brake(). Each specific car object will have values assigned to these properties, such as color = red, make = Toyota, model = Corolla.
The concept of classes and objects is one of the pillars of Object-Oriented Programming and forms the basis for all the other OOP principles. The best C++ tutorial will teach you how to create classes, define objects, and use methods and attributes effectively.
Step 2: Encapsulation in C++
Encapsulation refers to the bundling of data (attributes) and the methods (functions) that operate on the data into a single unit or class. This principle helps in hiding the internal state of an object from the outside world and only exposing the necessary parts.
In C++, encapsulation is implemented using access specifiers:
- public: Members are accessible from anywhere.
- private: Members are only accessible within the class itself.
- protected: Members are accessible within the class and its subclasses.
By using encapsulation, you ensure that the internal state of an object is protected from accidental or unauthorized modifications. Instead of directly accessing the attributes of an object, we use getter and setter methods to retrieve or modify the data. This helps in maintaining the integrity of the object’s state.
In this C++ tutorial point, you’ll learn how to define encapsulation and why it is essential for building clean and reliable code.
Step 3: Abstraction in C++
Abstraction involves hiding the complex details and only presenting the essential features of an object or system. The goal is to reduce complexity by exposing only the necessary parts of an object to the user.
In C++, abstraction is achieved using abstract classes and pure virtual functions. An abstract class is one that contains at least one pure virtual function. A pure virtual function is a function that is declared but not defined in the base class, forcing derived classes to provide their own implementation.
Abstraction allows you to focus on high-level concepts without getting bogged down in low-level details. It enables you to create modular and flexible programs, where the implementation details are hidden from the user but the essential functionality is available.
By reading through a best C++ tutorial, you can get a better grasp of how abstraction helps in designing systems where certain parts can evolve or change without affecting the overall design of the system.
Step 4: Inheritance in C++
Inheritance allows you to create a new class from an existing class. The new class, known as the derived class, inherits the attributes and methods of the base class. This promotes code reusability and establishes a relationship between the base and derived classes, often referred to as the "is-a" relationship.
For example, let’s say you have a base class Vehicle, and you create a derived class Car that inherits from Vehicle. The Car class will automatically have all the properties and behaviors of the Vehicle class but can also add its own unique features.
In C++, inheritance can be implemented with the public, protected, or private access specifiers. Public inheritance is the most commonly used, as it means that the derived class will inherit the public and protected members of the base class.
Inheritance helps in minimizing code duplication, as shared functionality can be written once in the base class and reused in multiple derived classes. This is one of the reasons OOP with C++ is so powerful.
Step 5: Polymorphism in C++
Polymorphism is the ability to present the same interface for different data types. In simpler terms, it allows different classes to provide their own unique implementation of the same method.
In C++, polymorphism is usually achieved through function overloading and function overriding.
- Function Overloading: This occurs when you define multiple functions with the same name but different parameters. The correct function is called based on the number and type of arguments passed.
- Function Overriding: This occurs when a derived class provides a specific implementation for a method that is already defined in the base class. The base class method is marked as virtual, and the derived class redefines it with the override keyword.
Polymorphism is a powerful feature that helps in achieving flexibility in your programs. It allows you to write code that works with objects of various types in a uniform manner.
Step 6: Putting It All Together
Now that we've covered the basic principles of Object-Oriented Programming in C++, let’s summarize the key takeaways:
- Classes and Objects: Classes define the blueprint for objects, while objects are instances of these classes.
- Encapsulation: Protects an object’s internal state by controlling access to its attributes and behaviors.
- Abstraction: Hides implementation details and exposes only the necessary information to the user.
- Inheritance: Allows you to create new classes based on existing ones, promoting code reuse.
- Polymorphism: Enables objects of different types to be treated as instances of the same class through common interfaces.
By mastering these concepts, you’ll be well on your way to writing efficient, maintainable, and scalable C++ programs. This C++ tutorial point has hopefully helped you understand the basics of OOP and how to apply them in C++.
Conclusion
Object-Oriented Programming is a fundamental concept that can greatly enhance your ability to write organized and reusable code in C++. Through the principles of encapsulation, abstraction, inheritance, and polymorphism, OOP enables you to design flexible and robust systems.
To master OOP in C++ tutorial, continue exploring tutorials, reading books, and practicing coding exercises. As you progress, you'll find that C++'s OOP features will help you solve increasingly complex problems in a structured and efficient way. Whether you are building a game, working on a software application, or writing system-level code, OOP in C++ is an essential skill that will serve you throughout your programming career.
What's Your Reaction?

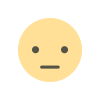

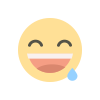


